Extract Specific Data From Pdf To Excel Using Python
As a data scientist, there are times when you need to extract specific data from a PDF file and store it in an Excel file. This can be a tedious and time-consuming task if you have to do it manually. Fortunately, Python provides an easy way to automate this process. In this article, we will show you how to extract specific data from PDF to Excel using Python.
Why extract data from PDF to Excel?
PDF files are widely used for document sharing, but they are not ideal for data analysis. Excel, on the other hand, is a powerful tool for data manipulation and analysis. By extracting data from PDF to Excel, you can easily analyze and manipulate the data to gain insights and make informed decisions.
For example, let's say you have a PDF file containing sales data that you need to analyze. By extracting the data and storing it in an Excel file, you can easily calculate the total sales, average sales, and other metrics to gain insights into your sales performance.
How to extract data from PDF to Excel using Python
Python provides several libraries for working with PDF files, but we will be using the PyPDF2 library for this tutorial. PyPDF2 is a pure-python library that allows you to manipulate PDF files.
Here are the steps to extract data from PDF to Excel using Python:
- Install PyPDF2 library - You can install the PyPDF2 library using pip by running the following command in your terminal:
pip install PyPDF2
- Import required libraries - You will need to import the following libraries in your Python script:
- PyPDF2
- pandas
- xlsxwriter
- re
- Read the PDF file - You can use the PyPDF2 library to read the PDF file and extract the text from it.
- Extract the data - You can use regular expressions to extract the specific data that you need from the text.
- Store the data in an Excel file - You can use the pandas library to store the data in an Excel file.
Example code
Here is an example Python script that extracts specific data from a PDF file and stores it in an Excel file:
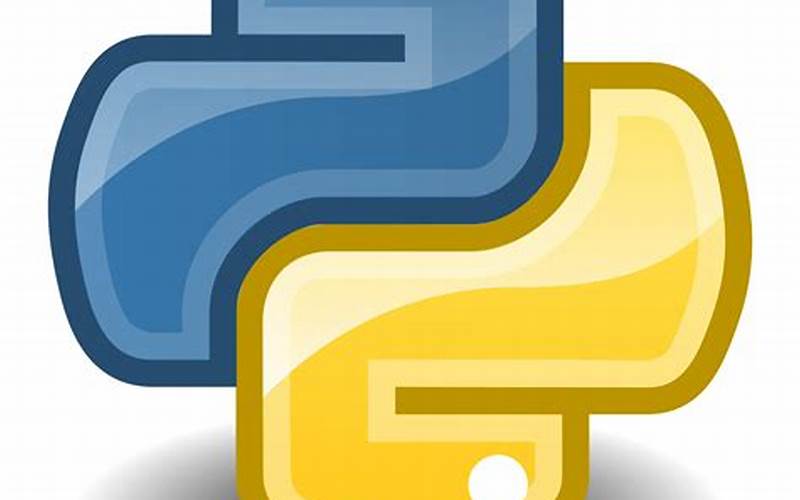
import PyPDF2import pandas as pdimport xlsxwriterimport re# Open the PDF filepdf_file = open('sample.pdf', 'rb')# Read the PDF file and extract the textpdf_reader = PyPDF2.PdfFileReader(pdf_file)text = ''for page in range(pdf_reader.getNumPages()):text += pdf_reader.getPage(page).extractText()# Extract the data using regular expressionsdata = []for line in text.split('\n'):if re.search('Total Sales:', line):data.append(re.findall('\d+', line)[0])elif re.search('Average Sales:', line):data.append(re.findall('\d+', line)[0])# Store the data in an Excel filedf = pd.DataFrame({'Metrics': ['Total Sales', 'Average Sales'],'Value': data})writer = pd.ExcelWriter('output.xlsx', engine='xlsxwriter')df.to_excel(writer, sheet_name='Sheet1', index=False)workbook = writer.bookworksheet = writer.sheets['Sheet1']worksheet.set_column('A:B', 15)writer.save()
Make sure to replace the file names and regular expressions with your own file names and patterns.
Conclusion
In this article, we have shown you how to extract specific data from a PDF file and store it in an Excel file using Python. Python provides an easy way to automate this process and save you time and effort. By using regular expressions, you can extract the specific data that you need and store it in a format that is easy to analyze and manipulate.
So next time you need to extract data from a PDF file, try using Python to automate the process and make your life easier.